微信设置
需要将我们电脑的微信设置为如下快捷方式,当然你也可以改其他的,不过需要该代码。
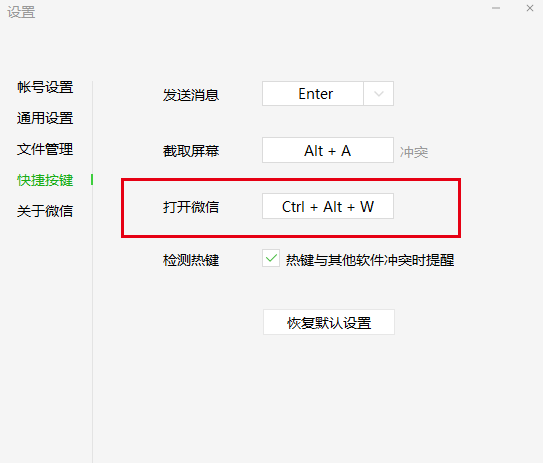
开发
为了方便操作我就不使用申请公众号开发的方式进行操作,直接模拟键盘操作控制微信。
编写一个小小的微信机器人
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88
| import java.awt.*; import java.awt.datatransfer.Clipboard; import java.awt.datatransfer.StringSelection; import java.awt.datatransfer.Transferable; import java.awt.event.KeyEvent;
public class WeChatRobot { private Robot bot = null; private Clipboard clip = null;
public WeChatRobot() { try { this.clip = Toolkit.getDefaultToolkit().getSystemClipboard(); this.bot = new Robot(); } catch (AWTException e) { e.printStackTrace(); } }
public void openWeChat() { bot.keyPress(KeyEvent.VK_CONTROL); bot.keyPress(KeyEvent.VK_ALT); bot.keyPress(KeyEvent.VK_W);
bot.keyRelease(KeyEvent.VK_CONTROL); bot.keyRelease(KeyEvent.VK_ALT);
bot.delay(1000); }
public void chooseFriends(String name) { Transferable text = new StringSelection(name); clip.setContents(text, null); bot.delay(1000); bot.keyPress(KeyEvent.VK_CONTROL); bot.keyPress(KeyEvent.VK_F); bot.keyRelease(KeyEvent.VK_CONTROL);
bot.delay(1000);
bot.keyPress(KeyEvent.VK_CONTROL); bot.keyPress(KeyEvent.VK_V); bot.keyRelease(KeyEvent.VK_CONTROL);
bot.delay(2000);
bot.keyPress(KeyEvent.VK_ENTER);
}
public void sendMessage(String message) { Transferable text = new StringSelection(message); clip.setContents(text, null); bot.delay(1000); bot.keyPress(KeyEvent.VK_CONTROL); bot.keyPress(KeyEvent.VK_V); bot.keyRelease(KeyEvent.VK_CONTROL); bot.delay(1000);
bot.keyPress(KeyEvent.VK_ENTER);
bot.delay(1000); bot.keyPress(KeyEvent.VK_CONTROL); bot.keyPress(KeyEvent.VK_ALT); bot.keyPress(KeyEvent.VK_W);
bot.keyRelease(KeyEvent.VK_CONTROL); bot.keyRelease(KeyEvent.VK_ALT); } }
|
编写一个发送的功能
我这里使用了两种发送方式,一种是定时延迟,一种是立即发送。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86
| import java.text.ParseException; import java.text.SimpleDateFormat; import java.util.Date; import java.util.concurrent.Executors; import java.util.concurrent.ScheduledExecutorService; import java.util.concurrent.TimeUnit;
public class StartSendMsgTask { private final WeChatRobot robot = new WeChatRobot(); ScheduledExecutorService exe = Executors.newSingleThreadScheduledExecutor();
public void sendMsgNow(String friendName, String message) { printLog(friendName, message); robot.openWeChat(); robot.chooseFriends(friendName); robot.sendMessage(message); }
public void sendMsgSchedule(String friendName, String timeStr, String message) { exe.schedule(() -> sendMsgNow(friendName, message), getDate(timeStr), TimeUnit.SECONDS); }
private void printLog(String friendName, String message) { SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss"); System.out.println("-----------------发送消息-----------------"); System.out.println("当前时间: " + sdf.format(new Date())); System.out.println("发送对象: " + friendName); System.out.println("发送内容: " + message); }
private long getDate(String timeStr) { SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd"); String currentDate = sdf.format(new Date()); String targetTime = currentDate + " " + timeStr;
sdf = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss"); long targetTimer = 0; try { targetTimer = sdf.parse(targetTime).getTime(); } catch (ParseException e) { e.printStackTrace(); } long currentTimer = System.currentTimeMillis(); if (targetTimer < currentTimer) { targetTimer += 1000 * 60 * 60 * 24; } Date date = new Date(targetTimer);
return (date.getTime() - System.currentTimeMillis()) / 1000; } }
|
最后我们就可以使用啦
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39
|
public class Main { public static void main(String[] args) { System.out.println("Start Send Message Task!"); String msg = "今天是2021年3月26日,星期五\n" + "\n" + "首先今天好也想你喔(づ ̄3 ̄)づ╭❤~,然后我就要来播送天气预报了!!\n" + "\n" + "今天最:高温 29.0℃,最低温 20.0℃\n" + "\n" + "多云,风力<3级,空气质量是良\n" + "\n" + "今天将在 18:39 太阳会缓缓落下,我会在家做好饭等你哟!\n" + "\n" + "双子座今日运势\n" + "\n" + "健康指数:92%\n" + "商谈指数:88%\n" + "幸运颜色:白色\n" + "幸运数字:8\n" + "\n" + "综合运势: 今天整体财运有点背,不太适合投资或是买乐透,建议可以做个理财计划,调整一下策略。中午将会花一些时间整理自己内心的小抽屉,其实也是时候用感性的思维清理那些烦人的问题了。\n" + "\n" + "最后阴晴之间,谨防紫外线侵扰\n" + "\n" + "爱你٩(๑>◡<๑)۶傻宝宝!!!";
StartSendMsgTask startSendMsgTask = new StartSendMsgTask(); startSendMsgTask.sendMsgNow("德玛西亚群", msg); startSendMsgTask.sendMsgSchedule("德玛西亚群", "11:08:30", msg); } }
|
从代码注释可以看出我们这里可以使用立刻发送或者定时发送,其中定时发送的时间如果超过了当前时间就会自动在明天发送。
效果
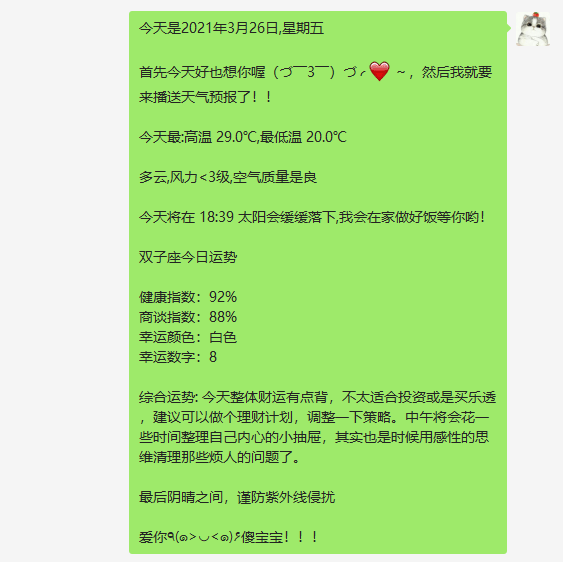
其他版本
以前写的邮件版本:https://github.com/HWYWL/love-mail